구조체 포인터 선언과 초기화
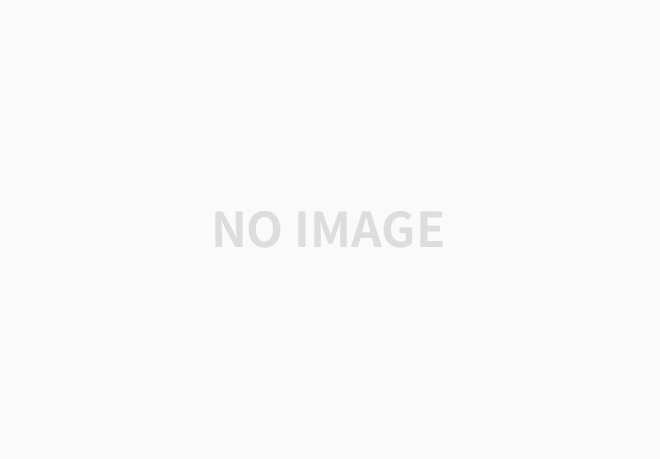
1. 구조체 포인터 선언
struct guy *him; /*구조체를 가리키는 포인터*/
새로운 구조체를 생성하지 않는다. 새로운 구조체를 생성하지 않지만, 구조체 포인터 자체 크기는 메모리에 할당된다. 기존의 guy형 구조체를 가리키도록 할 수 있다. barney이 guy 형의 구조체를 갖는다면, 아래와 같이 사용할 수 있다.
2. 구조체 포인터 초기화
him = &barney;
구조체 변수 선언 (struct book library;)을 하면 구조체 안 멤버들에게 기억 공간을 할당한다. 단순 구조체 설계는 메모리를 할당하지 않는다.
구조체의 멤버 가리키는 방법-2
1. -> 연산자 (arrow operator)
him-> income
Indirect member(ship) operator 이용. 포인터를 이용해 간접적으로 멤버에 접근하기 때문이다.
him: 포인터 변수
income: 포인터가 가리키는 구조체의 한 멤버
포인터 변수 -> 포인터가 가리키는 구조체의 한 멤버
him -> income의 자료형은 멤버의 자료형과 동일하다.
2. (*포인터변수).구조체멤버이름
him 포인터변수와 fellow[0] 구조체 변수가 같은 주소를 갖는다면(him == fellow[0]), 해당 주소를 갖는 변수가 저장하는 값(value)도 같다. (*him == fellow[0])
fellow[0].income == (*him).income /*주소가 동일한점을 이용하여 (*him)으로 대체하여 작성*/
참고
연산자 우선순위
dot(.) 연산자가 *연산자보다 우선순위가 높기 때문에 () 괄호 필요
him 구조체 포인터 변수가 가지는 주소값이 guy형 구조체인 barney 변수의 주소값과 같다면, 다음을 만족한다.
barney.income == (*him).income == him->income /* him == &barney 일 때*/
구조체 태그(tag, 이름)의 크기
구조체의 크기는 구조체 안에 선언한 자료형 크기의 합과 같다.
/*freind.c --구조체를 가리키는 포인터를 사용*/ #include <stdio.h> #define LEN 20 struct names { //총 40bytes char first[LEN]; //20bytes char last[LEN]; //20bytes }; struct guy { //총 84bytes struct names handle; // 40bytes char favfood[LEN]; //20bytes char job[LEN]; //20bytes float income; //4bytes }; /*초기화 부분은 생략. 전체 코드 참고*/ int main(void) { float a; printf("사이즈 names구조체: %d\n", sizeof(struct names)); printf("사이즈 guy구조체: %d\n", sizeof(struct guy)); printf("사이즈 float: %d\n", sizeof(a)); printf("사이즈 guy fellow[0]구조체: %d\n", sizeof(fellow[0])); printf("사이즈 guy fellow[1]구조체: %d\n", sizeof(fellow[1])); printf("사이즈 guy fellow구조체 변수: %d\n", sizeof(fellow)); return 0; }
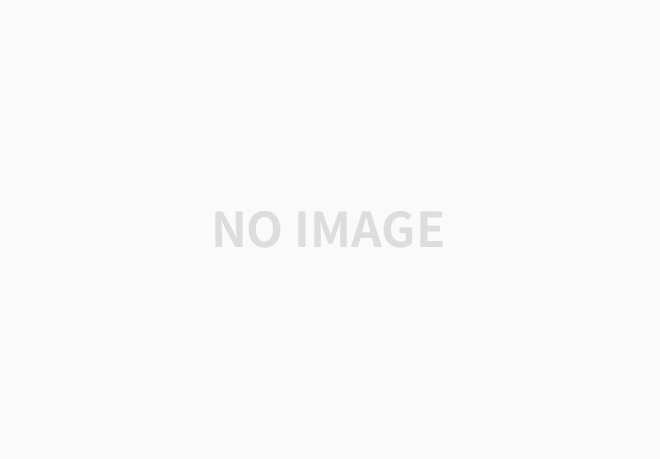
구조체 변수 선언 시 배열로 선언했다면(구조체의 배열), 해당 배열의 이름을 이용한 크기는 (배열의 크기 * 구조체 크기) bytes 이다. fellow는 구조체의 배열이다. 즉 fellow[0]이 구조체라는 것을 의미한다.
전체코드
/*freind.c --구조체를 가리키는 포인터를 사용*/ #include <stdio.h> #define LEN 20 struct names { char first[LEN]; char last[LEN]; }; struct guy { struct names handle; char favfood[LEN]; char job[LEN]; float income; }; int main(void) { struct guy fellow[2] = { {{"Ewen", "Villard"}, "grilled salmon", "personality coach", 80800.00 }, {{"Rodney", "Swillbelly"}, "tripe", "tabloid editor", 232400.00 } }; struct guy* him; /*구조체를 가리키는 포인터*/ printf("주소 #1 %p #2: %p\n", &fellow[0], &fellow[1]); him = &fellow[0]; /*포인터에게 가리킬 곳을 알려준다.*/ printf("포인터 #1: %p #2: %p\n", him, him + 1); printf("him->income은 $%.2f: (*him).income은 $%.2f\n", him->income, (*him).income); him++; //다음 구조체를 가리키게 한다. printf("him->favfodd는 %s: him->handle.last는 %s\n", him->favfood, him->handle.last); float a; printf("사이즈 names구조체: %d\n", sizeof(struct names)); printf("사이즈 guy구조체: %d\n", sizeof(struct guy)); printf("사이즈 float: %d\n", sizeof(a)); printf("사이즈 guy fellow[0]구조체: %d\n", sizeof(fellow[0])); printf("사이즈 guy fellow[1]구조체: %d\n", sizeof(fellow[1])); printf("사이즈 guy fellow구조체 변수: %d\n", sizeof(fellow)); printf("사이즈 him 구조체 포인터 변수: %d\n", sizeof(him)); return 0; }
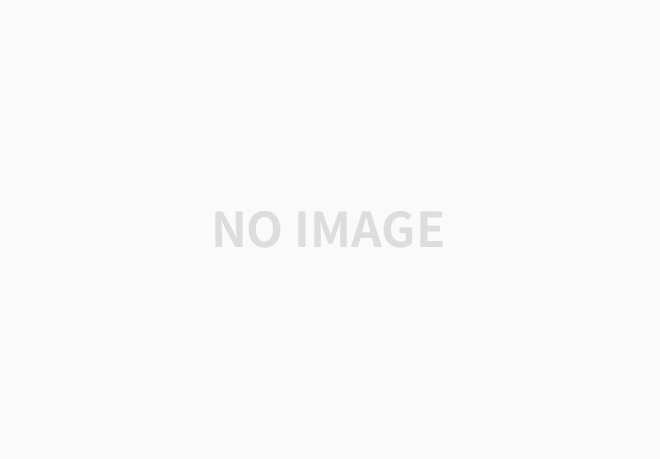
'C > 공부기록' 카테고리의 다른 글
[C언어] 함수 포인터 작성 방법, 사용 목적, 활용 정리 (0) | 2021.11.03 |
---|---|
[C언어] const와 포인터 활용 정리 (0) | 2021.09.20 |
[C기초 플러스] strcpy()함수 개념 및 기능 정리 (널 문자 복사) (0) | 2021.09.19 |
댓글